dependency injection using microsoft unity application block
Edit
In this article we will learn about implementing dependency injection using Microsoft Unity Application Block.
Application Blocks are classes distributed as Visual Studio project which you can download from Microsoft Website and add to your .Net Application. They are written by Microsoft's Patterns and Practices team. You can also use NuGet Packet Manager to add them to your project.
In case you are not familiar with Dependency Injection Pattern I would suggest you to read these two articles. inversion of control (IOC) design pattern & dependency injection design pattern. Knowing IOC is a prerequisite for learning Dependency Injection as Dependency Injection is just one of the techniques to implement IOC ( Inversion of Control )
How To Construct The Dependency ?
To construct dependencies of a class we have something called the Dependency Injection Container. The container is a map of dependencies and logic to create the dependencies which are required by your class. When you ask for dependency the container look for a dependency in the collection, sees if it's already created, if the dependency is already created it will return that dependency, otherwise, it will create it stored it, and then return the dependency. Here I am using a singleton for the above. The main advantage of using a container is it can resolve complex dependencies transparently. To Explain the Unity Application block I would use the same sample code from the previous article dependency injection design pattern and modify it to use Microsoft Application Block. This is what I am going to do. I would create a new project. Add Microsoft Unity Application block and copy-paste code from the previous article. Since the article was written on request by a fresher I would write in detail and use images as much as possible.
Setting up the application
Create a Console Application
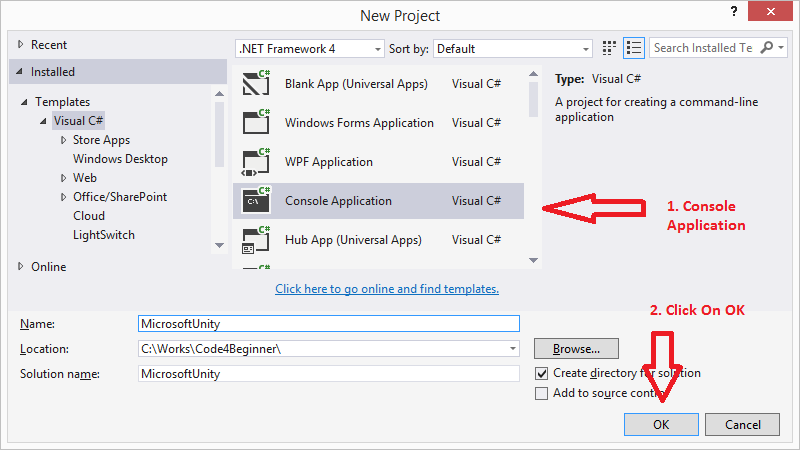
Add Microsoft Unity Application Block Using NuGet Package Manager
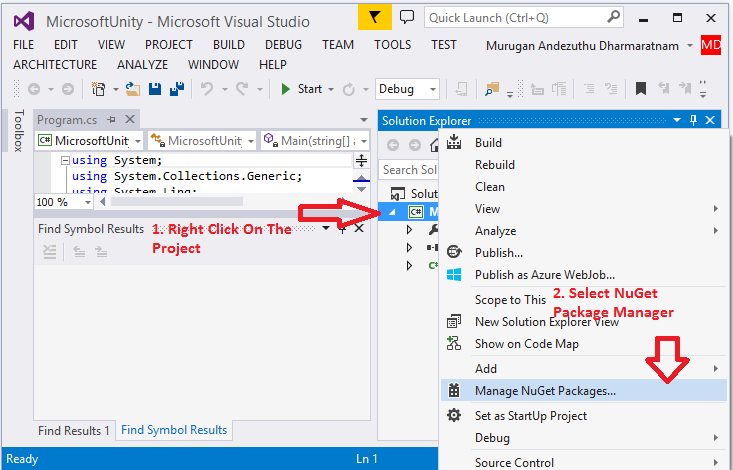
Installing the Package
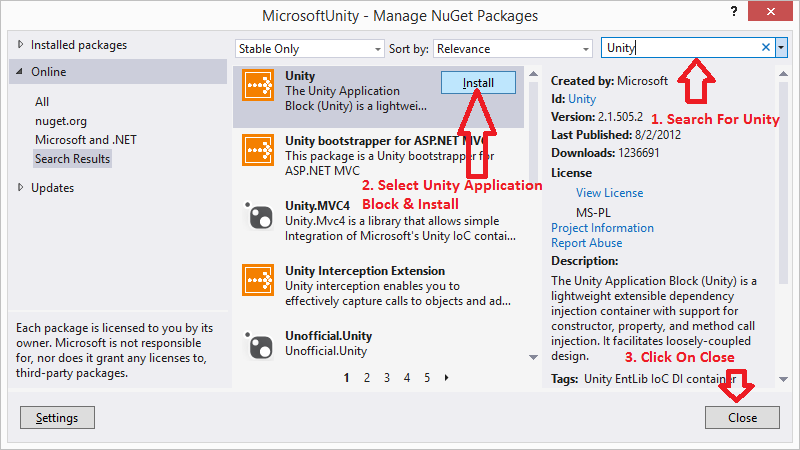
Code Not Using Unity
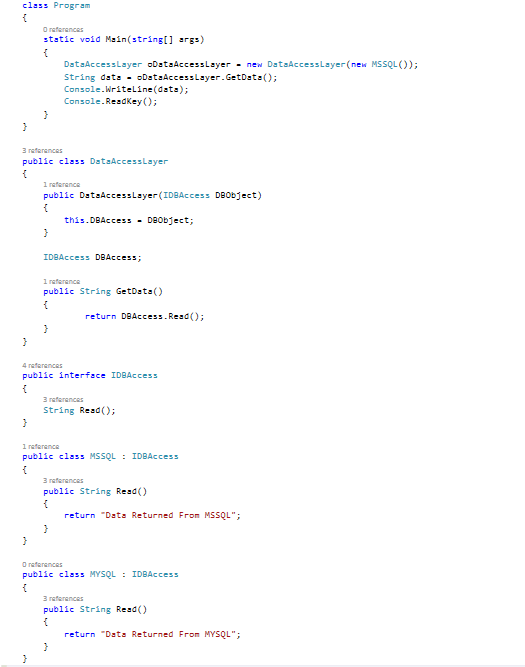
Output From Above Code
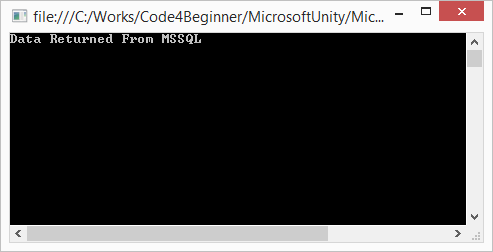
In this article, we are not much concerned about the output. I decided to put output just to let you know that its a working application
Unity Dependency Injection Components
To start coding using Microsoft Unity Application block its important to understand the different elements. Below are the list of key Methods used in Dependency Injection using Microsoft Unity.
- UnityContainer: Create a Unity Container
- Resolve: Returns an Object, resolve an instance of the default request type from the Continer.
- Register: Register a type mapping with the container. RegisterType tells the Container which class to use that implements the implement the interface.
- Dispose: Used to dispose the container reference.
- LifetimeManager: In Unity container Lifetime Manager is used by the Unity container to manages lifetime resolution of the objects and also manage the creation.
Now we will modify the code to user Unity Container to create Objects instead of explicit calls to class constructors and property setters.
To Start using Unity we need to add namespace using Microsoft.Practices.Unity, create a Unity Container, and create an object using the container.Resolve
Let us first create an instance of MSSQL using Unity and display the value from the Read Method.
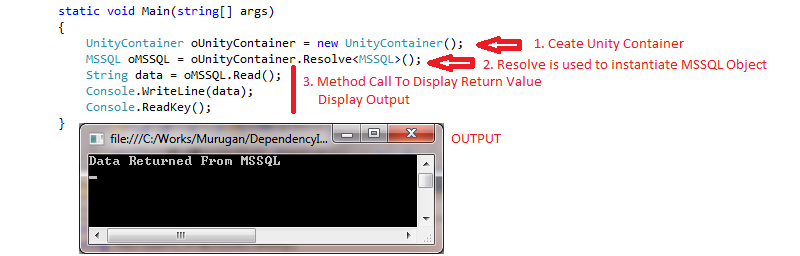
The program displays "Data Returned from MSSQL", This is the simplest Hello World Type example you can write in Microsoft Unity Application Block.
Unity Using Interface
Let us modify the Above program to use interfaces. To use an Interface we need to use the RegisterType Method. An interface contains only the signatures, RegisterType tells the Container which class to use that implements the implement the interface. Unity will instantiate an object from the specified class ( MSSQL or MYSQL ) when we resolve any object containing a dependency of Interface (eg IDBAccess ) type.
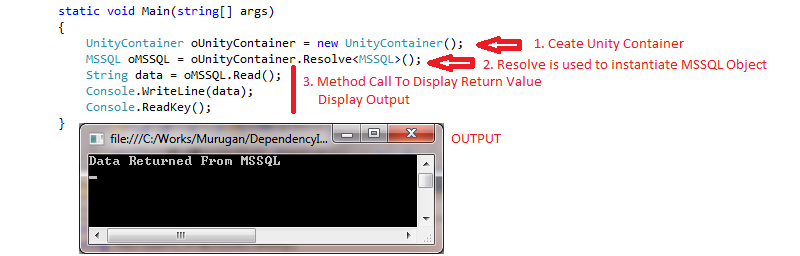
Lifetime Manager
When you register the type of an existing object you specify the lifetime of the Object or the default lifetime is used. The Unity container manages the creation and resolution of objects based on a lifetime you specify when you register the type of an existing object.
Type of Lifetime Managers
- TransientLifetimeManager: Unity creates and returns a new instance of the requested type for every call to the Resolve or ResolveAll method
- ContainerControlledLifetimeManager: ContainerControlledLifetimeManager implements a singleton behavior for objects, First time a call is made it creates an Instance, for all subsiquent calls it returns the same Instance of the registered Type each time you call the Resolve or ResolveAll method.
- HierarchicalLifetimeManager: ContainerControlledLifetimeManager, Unity returns the same instance of the registered type or object each time you call the Resolve or ResolveAll method or when the dependency mechanism injects instances into other classes.The distinction is that when there are child containers, each child resolves its own instance of the object and does not share one with the parent. When resolving in the parent, the behavior is like a container controlled lifetime; when resolving the parent and the child you have different instances with each acting as a container-controlled lifetime. If you have multiple children, each will resolve its own instance.
- PerThreadLifetimeManager: For this lifetime manager the behavior is like a TransientLifetimeManager, but also provides a signal to the default build plan, marking the type so that instances are reused across the build-up object graph.
- ExternallyControlledLifetimeManager: The ExternallyControlledLifetimeManager class provides generic support for externally managed lifetimes. This lifetime manager allows you to register type mappings and existing objects with the container so that it maintains only a weak reference to the objects it creates when you call the Resolve or ResolveAll method or when the dependency mechanism injects instances into other classes based on attributes or constructor parameters within that class. This allows other code to maintain the object in memory or dispose it and enables you to maintain control of the lifetime of existing objects or allow some other mechanism to control the lifetime. Using the ExternallyControlledLifetimeManager enables you to create your own custom lifetime managers for specific scenarios. Unity returns the same instance of the registered type or object each time you call the Resolve or ResolveAll method or when the dependency mechanism injects instances into other classes. However, since the container does not hold onto a strong reference to the object after it creates it, the garbage collector can dispose of the object if no other code is holding a strong reference to it.