8086 program to convert hexadecimal to ascii
Edit
8086 assembly language program to convert from hex or decimal to ASCII. Ideally what you are trying to do is to display a number as a numeric character. In this program, we will display decimal 356 or hex 164 as a string 250
The first thing to do would be to run the program.
8086 code hex or decimal to ASCII
;---------------------------------------- ;Program : clear screen ;FileName : hextoasc.asm ;I/P : number (365 decimal) in ax ;O/P : displays 365 ;---------------------------------------- .model tiny .code org 100h main proc near mov ax,365d mov si,offset message mov cx,00h mov bx,0ah hexloop1: mov dx,0 div bx add dl,'0' push dx inc cx cmp ax,0ah jge hexloop1 add al,'0' mov [si],al hexloop2: pop ax inc si mov [si],al loop hexloop2 inc si mov [si],'$' mov ah,09h mov dx,offset message int 21h mov ah,4ch mov al,00 int 21h endp message db 10 dup(?) end main
Output
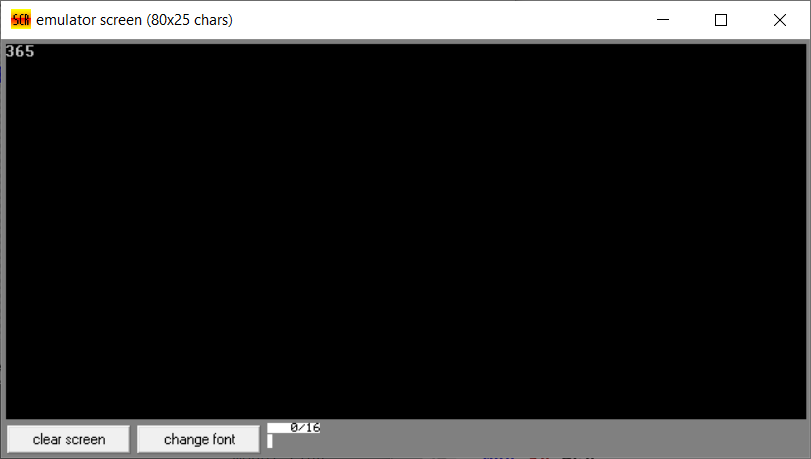
I am using emu8086 to run the program. You can also run the program using tasm or masm
Generate executable using
tasm filename.asm tlink /t filename
let's improve the code a little bit. The output will be the same. I am going to modify the code to display two numbers instead of one. For this the main thing, I have to do is to write a procedure for converting from hexadecimal to ASCII. Check the improved code below
Improved code
;---------------------------------------- ;Program : clear screen ;FileName : hextoasc.asm ;I/P : number (365 decimal) in ax & number (969 decimal) in ax ;O/P : displays 365 & 969 ;---------------------------------------- .model tiny .code org 100h main proc near mov ax,356d mov si,offset message call hexToAsc mov ah,09h mov dx,offset message int 21h mov ah,09h mov dx,offset newline int 21h mov ax,969d mov si,offset message call hexToAsc mov ah,09h mov dx,offset message int 21h mov ah,4ch mov al,00 int 21h endp newline db 0ah,0dh,'$' message db 10 dup(?) value dw 0 hexToAsc proc near ;AX input , si point result storage addres mov cx,00h mov bx,0ah hexloop1: mov dx,0 div bx add dl,'0' push dx inc cx cmp ax,0ah jge hexloop1 add al,'0' mov [si],al hexloop2: pop ax inc si mov [si],al loop hexloop2 inc si mov [si],'$' ret endp end main
Output
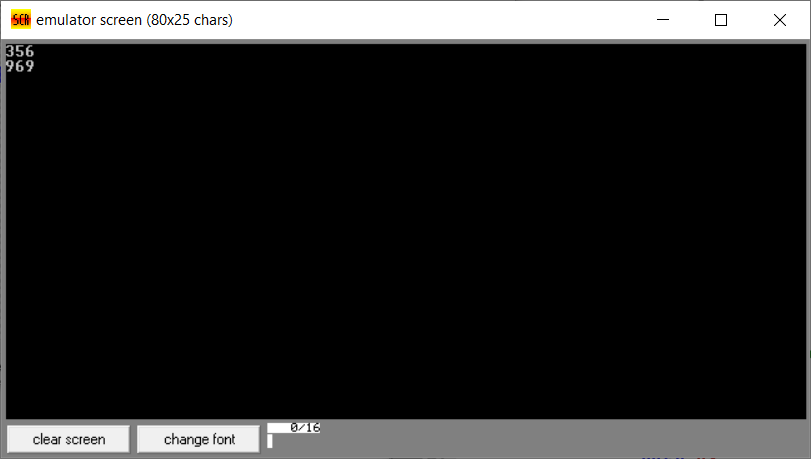