asp.net mvc create an autocomplete textbox using jquery autocomplete
Edit
In this article, we will look at how to autocomplete textbox in asp.net MVC with the database using c# and jquery. This is what we are trying to do, In the textbox when the user types in some words the autocomplete will provide us with some suggestions. The final output will look like the screen below.
Output
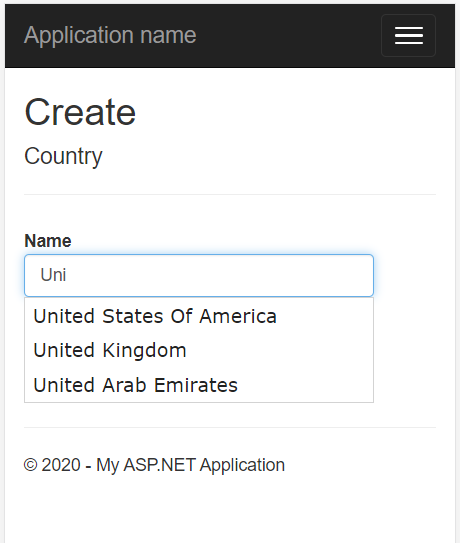
Autofill from a List
I have a list of countries & when the user types in country name autofill will display the suggestions. First, we will write code for an autofill textbox from a hardcoded list then we will modify the code to get the data from a database.
First, create an asp .net MVC application. then let's create a Country class in the Models folder. The class will have two properties Id and Name.
public partial class Country { public int Id { get; set; } public String Name { get; set; } }
Now we will add one method to the class which returns the hardcoded list of countries.
public ListGet() { List<country> lstCountry = new List<country>() { new Country() { Id = 91, Name="India" }, new Country() { Id = 1, Name="United States Of America" }, new Country() { Id = 1, Name="United Kingdom" }, new Country() { Id = 1, Name="United Arab Emirates" }, new Country() { Id = 7, Name="Russia" }, new Country() { Id = 13, Name="Australia" }, new Country() { Id = 14, Name="Austria" }, new Country() { Id = 17, Name="Bahrain" }, new Country() { Id = 18, Name="Bangladesh" }, new Country() { Id = 21, Name="Belgium" }, new Country() { Id = 24, Name="Bermuda" }, new Country() { Id = 25, Name="Bhutan" }, new Country() { Id = 33, Name="Bulgaria" }, new Country() { Id = 35, Name="Burundi" }, new Country() { Id = 36, Name="Cambodia" }, new Country() { Id = 38, Name="Canada" }, new Country() { Id = 43, Name="Chile" }, new Country() { Id = 44, Name="China" }, new Country() { Id = 206, Name="Sri Lanka" }, new Country() { Id = 210, Name="Swaziland" }, new Country() { Id = 211, Name="Sweden" }, new Country() { Id = 212, Name="Switzerland" }, new Country() { Id = 213, Name="Syria" }, new Country() { Id = 214, Name="Taiwan" } }; return lstCountry; }
Create Countries Controller
- Right click on the Controller folder and select Add -> Controller.
- Select MVC Controller with read write actions.
- Click on add and name the controller CountriesController
Add Create View
- Open the Countries Controller
- Right click on Countries/Create Action method
- Select Add View
- In the template select Create
- Model Class Select Country
- Click on Add
Add GetCountries Method to the Countries Controller
// GET: Countries/Create [HttpGet] public JsonResult GetCountries(String filter) { filter = filter ?? ""; ListCountries = new Country().Get(); var retval = Countries.Where(x => x.Name.Contains(filter)); return Json(retval, JsonRequestBehavior.AllowGet); ; }
Add autofill javascript code to the view
<script type="text/javascript"> function getdata() { var txt = $("#Name").val(); var url = "/Countries/GetCountries/?filter=" txt; $("#Name").autocomplete({ source: function (request, response) { $.ajax({ url: url, method: "GET", dataType: "json", success: function (data) { response($.map(data, function (item) { return { label: item.Name, value: item.Name }; })) } }) } }); } $("#Name").keyup(function () { console.log("hello world"); getdata(); }); </script>
$("#Name") the name should be replaced with Id of which ever textbox to which you want to add the autocomplete functionality
Exception Errors
You might encounter some errors and drop-down might not work. Autocomplete uses jquery UI, so make sure the below files are added right after jquery.
<script src="//code.jquery.com/ui/1.12.1/jquery-ui.js"></script> <link rel="stylesheet" href="//code.jquery.com/ui/1.12.1/themes/smoothness/jquery-ui.css">
If you are still facing issues check this link jquery error autocomplete is not a function